A JavaScript library for building user interfaces
A Simple Component
React components implement a render()
method that takes input data and returns what to display. This example uses an XML-like syntax called JSX. Input data that is passed into the component can be accessed by render()
via this.props
.
A Stateful Component
In addition to taking input data (accessed via this.props
), a component can maintain internal state data (accessed via this.state
). When a component’s state data changes, the rendered markup will be updated by re-invoking render()
.
An Application
Using props and state, we can put together a small Todo application. This example uses state to track the current list of items as well as the text that the user has entered. Although event handlers appear to be rendered inline, they will be collected and implemented using event delegation.
A Component Using External Plugins
React allows you to interface with other libraries and frameworks. This example uses remarkable, an external Markdown library, to convert the textarea’s value in real time.
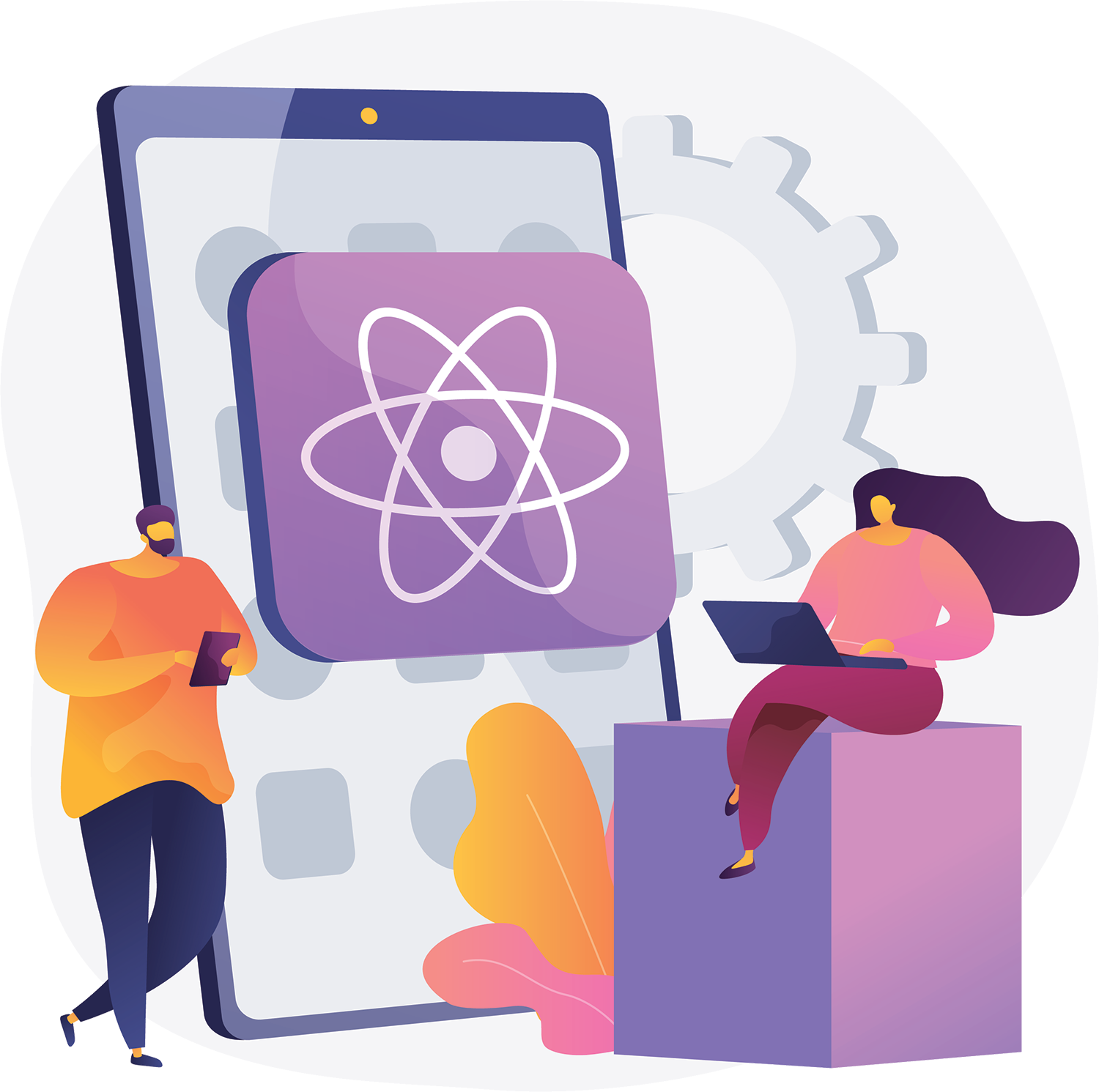
Declarative
React is a JavaScript library for building user interfaces. It helps create interactive and declarative views. Declarative code is easier to debug and improves app performance.
Component-Based
React allows you to build modular, reusable components that manage their own state. These components can be composed to create complex UIs. Logic is written in JavaScript, making it easy to pass rich data through the app and keep state out of the DOM.
Learn Once, Write Anywhere
React is flexible and can be used with a variety of technology stacks. It allows for the development of new features without rewriting existing code. React can also be rendered on the server and used to power mobile apps.